SharePoint 2013 Apps using JavaScript Object Model (JSOM)
Referred Link - http://www.dotnetcurry.com/sharepoint/1028/sharepoint-apps-javascript-object-model-jsom
Note:
- SharePoint App can be created on the Developer Site
- The default Administrator cannot create App. So there should be other users with Administrator credentials in the Active Directory
- This user now must be the Administrator user group of the Developer site
Some important points regarding SharePoint App
When we work on SharePoint Apps, we come across two major concepts: App Web and Host Web.
App Web - App is required to access SharePoint components like Lists, WorkFlow types, Pages, etc. so we need a separate site where these components are deployed. This site is called as App Web.
Host Web - This is the SharePoint site where the App is actually installed, it is called as Host web.
Detailed information for App Web and Host web can be found from here: http://msdn.microsoft.com/en-us/library/office/fp179925.aspx
In the following steps, we will see the implementation of a SharePoint 2013 App
Step 1: Open SharePoint 2013 Developer Site and create a List App with the following Fields:
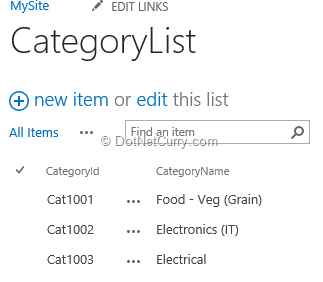
Note: When we create a List in SharePoint, the default field of name ‘Title’ is already available. Rename the Title field to CategoryId. But when we write the code, we need to refer this field using ‘Title’ and not as CategoryId.
Step 2: Open Visual Studio 2013 and create a new SharePoint App as shown here:
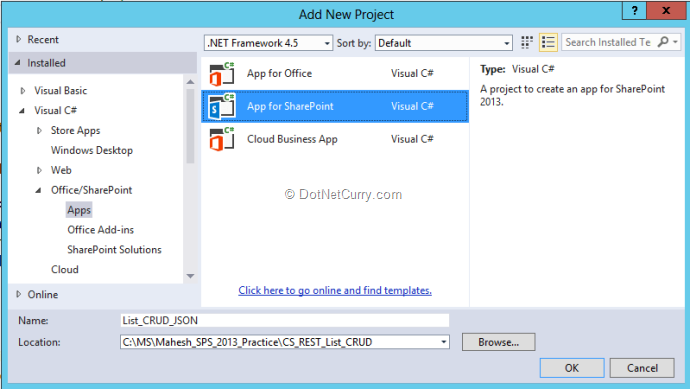
Step 3: Open the Default.aspx from the ‘Pages’ folder and in the asp.Content with id as PlaceHolderMain, add the below HTML markup code:
Step 4: Since we are creating a SharePoint App, the complete code will be written using JavaScript. In SharePoint 2013 we are provided with the JavaScript Object Model (JSOM), so we need to understand some of the main objects which are usable for App development. In the project, we have _references.js file under the Scripts folder which contains necessary references for JavaScript files for App development. In this case we will be using SP.ClientContext object.
Sp.ClientContext
- Represents the SharePoint Objects and Operations context.
- Used to access SiteCollection, WebSite, List, etc.
- Used to perform async calls to the SharePoint to accessing data.
- Load method: Retrieves the properties of a client object from the server.
- executeQueryAsync method: Executes the current pending request asynchronously on the server.
Step 5: Open App.js from the Scripts folder and add the JavaScript code for performing CRUD operations on the CategoryList. There are some specific steps we need to implement for every operation:
We need to work with the App Web and Host Web URL. To do that the following helper method with querystringparameter will help us out:
In the App.js declare variables to store the Host web and App web:
Get the Host Web and App Web Url in document.ready:
In the above code: SPHostUrl represents the full URL of the host site and SPAppWebUrl represents the full URL of the app web.
Declare the following global object for the current object context for SharePoint:
For performing operations using JSOM, we need to implement the following logic:
The above steps are commonly used across each of the methods.
Add the following method in the App.js for Loading List items:
" |
+ currentListItem.get_item(
'Title'
) +
"
+ currentListItem.get_item(
'CategoryName'
)+
"
";
}
table.html(innerHtml);
}),
Function.createDelegate(
this
, fail)
);
}
The above code performs the following operations:
- Use SP.CamlQuery() to create query object for querying the List
- The query object is set with the criteria using xml expression using set_viewXml() method
- Using getItems() method of the List the query will be processed
- executeQueryAsync() methods processes the batch on the server and retrieve the List data. This data is displayed using HTML table after performing iterations on the retrieved data
Add the following method in App.js to create a new list entry:
The above code performs the below operations:
- To add a new item in the list, the SP.ListCreationInformation() object is used
- This object is then passed to the addItem() method of the List. This method returns the ListItem object
- Using the set_item() method of the ListItem the values for each field in the List is set and finally the list is updated
In App.js, add the following code to search the items for the list based upon it:
Now add the following code to Update the ListItem based upon the id:
In the above two methods, the implementation is almost similar. The only difference is that in the updateItem() method after searching the record, the new value is set for the CategoryName and the ListItem is updated.
In App.js add the below code to Delete ListItem:
This code too is similar with the updateItem() method, only difference is that after searching the ListItem based upon the id, the ‘deleteObject()’ method is called on ListItem object.
Now add the following two methods for Callback in App.js
Call the ‘listAllCategories()’ method in document.ready() and call the above methods in the HTML buttons’ click event as below:
Step 6: Since the App makes call to the SharePoint List, we need to set permission for the App to access list. To set these permissions, in the project double-click on AppManifest.xml and from the permission tab set the permissions as below:
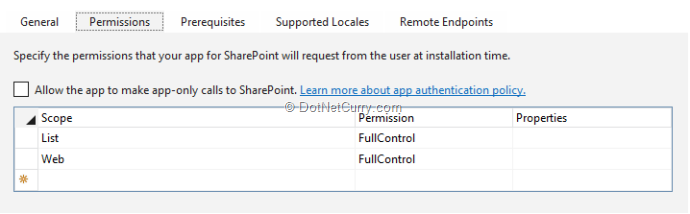
Step 7: Build the project and make sure that it is error free. To deploy the App, right click on the project name and select the Option deploy. Once the deployment is successful, since we have defined the permissions for the App, we will be asked to Trust the App, this is shown here:
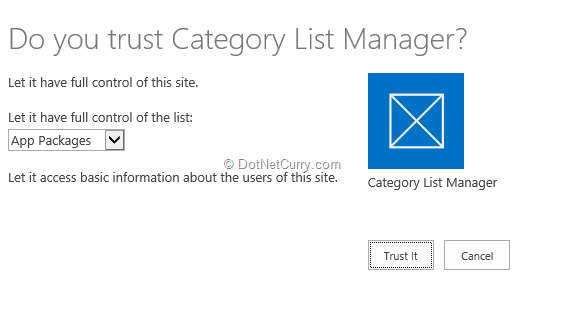
After clicking on ‘Trust it’, the browser will show the login where the credential information needs to be entered. Now the App will be displayed as follows:: (Since I already had data it is showing some values)
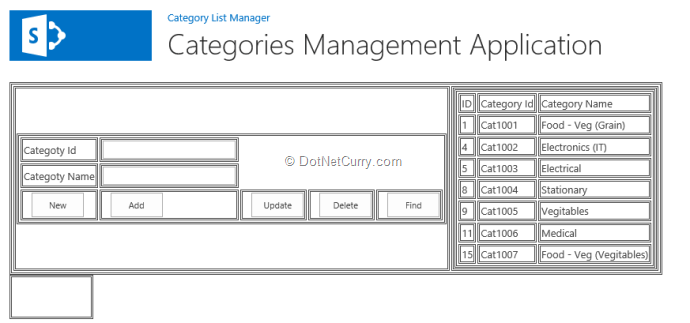
(Note: The project focuses on the JSOM coding and permission part, so pardon the HTML UI and my design skills)
To Search the record, click on the ‘Find’ button which brings up a JavaScript Prompt. Enter Item Id in it from the ID column of the table.
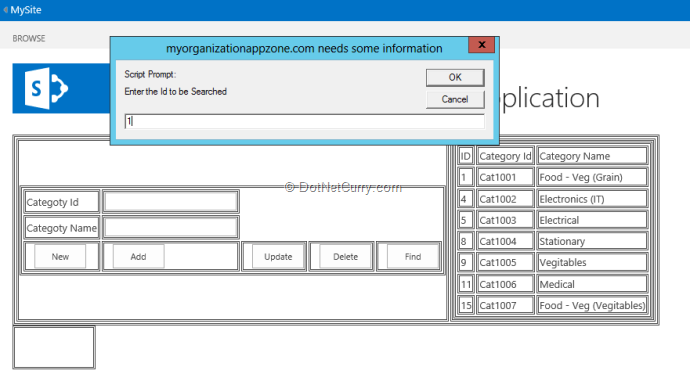
Click on ‘OK’, the record will be displayed in the TextBoxes as below:
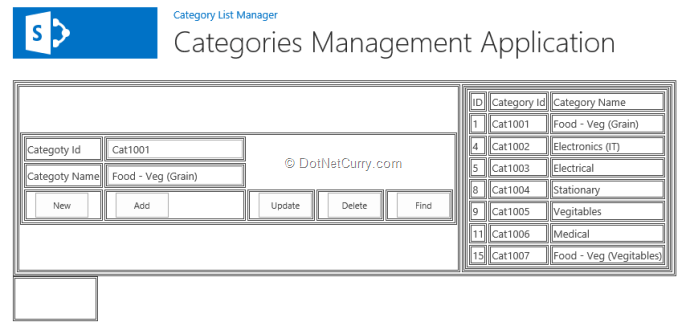
Now this record can be Updated and Deleted by using corresponding buttons.
Conclusion: Using JSOM in SharePoint 2013, an App part can be easily created. Using App Model, a developer can make use of his or her own skills of JavaScript and study of JavaScript API to manage the code for App in SharePoint.
0 comments